Snake typing games are a fun game to make as it doesn’t require a lot of code This is a basic implementation of the snake game, but it’s missing a few things intentionally and they’re left as further exploration for the reader.
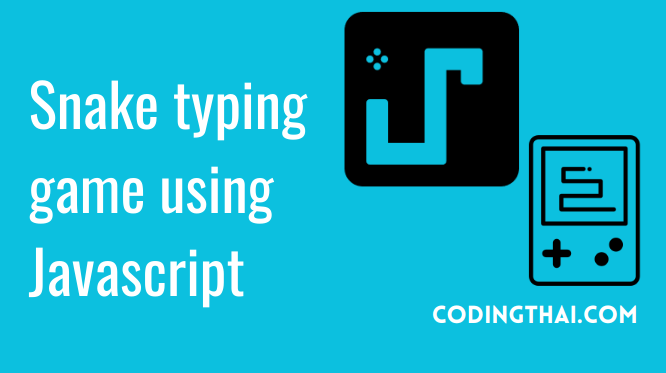
In this blog post, I’ll guide you through the steps of creating your own Snake typing Game using Javascript. You can play this game on a PC using keyboard arrow keys.
Language Used | HTML, CSS and Pure Javascript |
External Link / Dependencies | No |
Responsive | Yes |
You might like this
- Coder Animation Using HTML5 & CSS3
- SVG Text Animation Using HTML5 & CSS3
- Content Placeholder Using HTML5 CSS3 & JavaScript
- Enjoy Code Animation Using HTML5 & CSS3
There are 3 types of styles to connect CSS with HTML files. Inline CSS, Internal CSS, External CSS. For Inline CSS in this, we have to write the CSS code inside the HTML code using style Attribute elements. For internal CSS we have to use the Style tag in the Head section on HTML File. We have used this Internal CSS in The below section. Last is External CSS for this we have to create another CSS File in the same folder this
Preview of Snake typing game using Javascript
In this preview, we have used internal CSS in the code. In the internal CSS, we have to write the code in the head section using the Style tag. We have to write the code in <Style> CSS code </style> in the Head section in the HTML file. preview in codepen
See the Pen Untitled by Coding thai (@Codingthai) on CodePen.
Snake typing game using Javascript [Source code]
For Creating a Snake typing game using Javascript. First, you have to create three files (HTML, CSS & Javascript) files with the named index.html, style.css, and script.js in the same folder and you have to link the CSS and javascript files to HTML. after that paste, the HTML code in index.html, paste the CSS code in style.css and lastly paste the Javascript Code in Script.js that’s all after pasting the code.
First, you have to create an HTML file with the named index.html and paste the below HTML code on it and save it. Remember to give a .html extension to the HTML file.
<!DOCTYPE html> <html> <head> <title>Snake game codingthai</title> </head> <body> <canvas width="400" height="400" id="game"></canvas> </html>
After pasting the HTML code, Now have to create a second CSS file with the named style.css. Paste the below code on it and save it. Again remember to give a .css extension to the CSS file.
<style> body { height: 100%; margin: 0; } body { background:black; display: flex; align-items: center; justify-content: center; } canvas { background: blue; border: 5px solid white; } <\style>
After pasting the HTML and CSS code, Now have to create a third Javascript file with the named script.js. Paste the below code on it and save it. Again remember to give a .js extension to the javascript file.
<script> var canvas = document.getElementById('game'); var context = canvas.getContext('2d'); var grid = 16; var count = 0; var snake = { x: 160, y: 160, // snake velocity. moves one grid length every frame in either the x or y direction dx: grid, dy: 0, // keep track of all grids the snake body occupies cells: [], // length of the snake. grows when eating an apple maxCells: 4 }; var apple = { x: 320, y: 320 }; // get random whole numbers in a specific range // @see https://stackoverflow.com/a/1527820/2124254 function getRandomInt(min, max) { return Math.floor(Math.random() * (max - min)) + min; } // game loop function loop() { requestAnimationFrame(loop); // slow game loop to 15 fps instead of 60 (60/15 = 4) if (++count < 4) { return; } count = 0; context.clearRect(0,0,canvas.width,canvas.height); // move snake by it's velocity snake.x += snake.dx; snake.y += snake.dy; // wrap snake position horizontally on edge of screen if (snake.x < 0) { snake.x = canvas.width - grid; } else if (snake.x >= canvas.width) { snake.x = 0; } // wrap snake position vertically on edge of screen if (snake.y < 0) { snake.y = canvas.height - grid; } else if (snake.y >= canvas.height) { snake.y = 0; } // keep track of where snake has been. front of the array is always the head snake.cells.unshift({x: snake.x, y: snake.y}); // remove cells as we move away from them if (snake.cells.length > snake.maxCells) { snake.cells.pop(); } // draw apple context.fillStyle = 'yellow'; context.fillRect(apple.x, apple.y, grid-1, grid-1); // draw snake one cell at a time context.fillStyle = 'white'; snake.cells.forEach(function(cell, index) { // drawing 1 px smaller than the grid creates a grid effect in the snake body so you can see how long it is context.fillRect(cell.x, cell.y, grid-1, grid-1); // snake ate apple if (cell.x === apple.x && cell.y === apple.y) { snake.maxCells++; // canvas is 400x400 which is 25x25 grids apple.x = getRandomInt(0, 25) * grid; apple.y = getRandomInt(0, 25) * grid; } // check collision with all cells after this one (modified bubble sort) for (var i = index + 1; i < snake.cells.length; i++) { // snake occupies same space as a body part. reset game if (cell.x === snake.cells[i].x && cell.y === snake.cells[i].y) { snake.x = 160; snake.y = 160; snake.cells = []; snake.maxCells = 4; snake.dx = grid; snake.dy = 0; apple.x = getRandomInt(0, 25) * grid; apple.y = getRandomInt(0, 25) * grid; } } }); } // listen to keyboard events to move the snake document.addEventListener('keydown', function(e) { // prevent snake from backtracking on itself by checking that it's // not already moving on the same axis (pressing left while moving // left won't do anything, and pressing right while moving left // shouldn't let you collide with your own body) // left arrow key if (e.which === 37 && snake.dx === 0) { snake.dx = -grid; snake.dy = 0; } // up arrow key else if (e.which === 38 && snake.dy === 0) { snake.dy = -grid; snake.dx = 0; } // right arrow key else if (e.which === 39 && snake.dx === 0) { snake.dx = grid; snake.dy = 0; } // down arrow key else if (e.which === 40 && snake.dy === 0) { snake.dy = grid; snake.dx = 0; } }); // start the game requestAnimationFrame(loop); <\script>
That’s all after pasting the code your code will be successfully run. If you get any kind of error/problem in the code just comment or contact me on social media