Hello, learner today In this blog post, We will be creating a Typing Test App using Javascript. In the past post, we have created many projects one of them is the Aspect Ratio Calculator. Without wasting time Now it is time to create a Typing Test App.
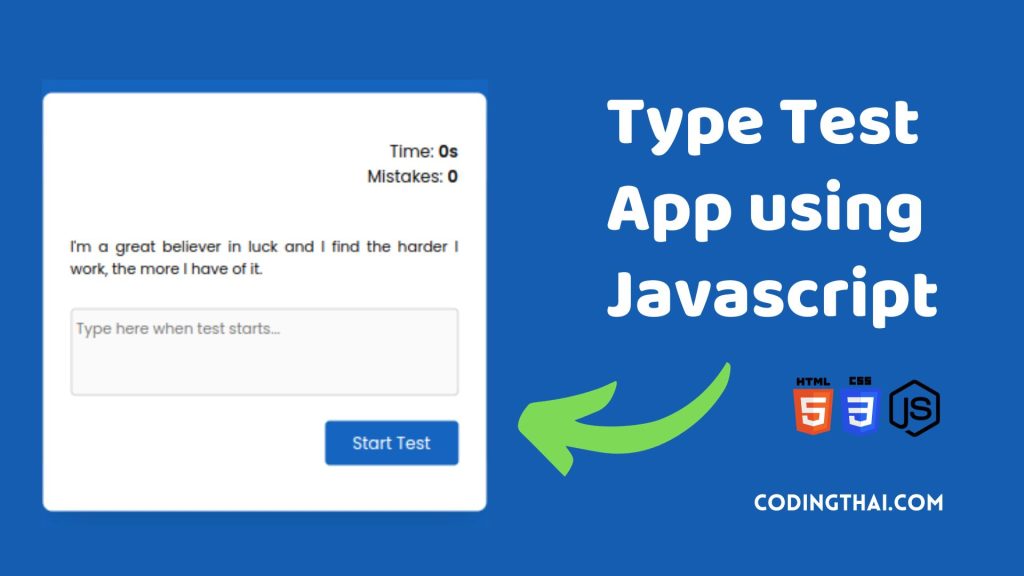
A typing test App is used to check how fast the user can type the word in a given amount of time. We will be Creating a typing Test App using JavaScript that presents a simple typing challenge and finds the performance of typing by calculating the Characters Per Minute (CPM), Words Per Minute (WPM) and the accuracy of the typed characters.
The Main Logic of the App is defined in a JavaScript file. Several functions work together to run the Typing test app. you can see all the functions in the Javascript section below.
As you see in the above output image. There is a typing test at first there is the given text you have to write in the below section. There you you see the time and Mistake at the top of the app. After you complete the text written you will get your result how much is your speed and accuracy of typing?
If you’re having difficulty understanding what I’m saying or what this Typing Test App then You can ask me in the comment.
Code By | Coding thai |
Language Used | HTML, CSS and JS |
Responsive | Yes |
External Link / Dependencies | No |
There are 3 types of styles to connect CSS with HTML files. Inline CSS, Internal CSS, External CSS. For Inline CSS in this, we have to write the CSS code inside the HTML code using style Attribute elements. For internal CSS we have to use the Style tag in the Head section on HTML File. We have used this Internal CSS in The below section. Last is External CSS for this we have to create another CSS File in the same folder this
Preview of Typing Test App
In this preview, we have used internal CSS in the code. In the internal CSS, we have to write the code in the head section using the Style tag. We have to write the code in <Style> CSS code </style> in the Head section in the HTML file. This code is run in Codepen.io
See the Pen Untitled by Coding thai (@Codingthai) on CodePen.
You might like this
Typing Test App using Javascript [Source code]
For Creating A Typing Test App using HTML, CSS, and JS. First, you have to create Three files (HTML, CSS, and JS) files with the named index.html, style.css, and Script.js in the same folder and you have to link the CSS and JS files to HTML. after that paste the below code, the HTML code in index.html, and paste the CSS code in style.css Again paste the Js code in script.js that’s all after pasting the code.
First, you have to create an HTML file with the named index.html paste the below HTML code on it, and save it. Remember to give a .html extension to the HTML file.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="style.css"> <title>Typing Test App | Codingthai</title> </head> <body> <div class="container"> <div class="stats"> <p>Time: <span id="timer">0s</span></p> <p>Mistakes: <span id="mistakes">0</span></p> </div> <div id="quote" onmousedown="return false" onselectstart="return false"></div> <textarea id="quote-input" rows="3" placeholder="Type here when test starts..."></textarea> <button id="start-test" onclick="startTest()">Start Test</button> <button id="stop-test" onclick="displayResult()">Stop Test</button> <div class="result"> <h3>Result</h3> <div class="wrapper"> <p>Accuracy: <span id="accuracy"></span></p> <p>Speed: <span id="wpm"></span></p> </div> </div> </div> <script src="index.js"></script> </body> </html>
After pasting the HTML code, Now have to create a second CSS file with the named style.css. Paste the below code on it and save it. Again remember to give a .css extension to the CSS file.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600;700&display=swap'); *{ margin: 0; padding: 0; box-sizing: border-box; font-family: 'Poppins', sans-serif; } body{ background-color: #1565c0; } .container{ width: 80vmin; padding: 50px 30px; background-color: #fff; position: absolute; transform: translate(-50%, -50%); top: 50%; left: 50%; border-radius: 10px; box-shadow: 0 20px 40px rgba(0, 0, 0, 0.15); } .stats{ text-align: right; font-size: 18px; margin-bottom: 30px; } .stats span{ font-weight: 600; } #quote{ text-align: justify; margin: 50px 0 30px 0; } textarea{ resize: none; width: 100%; border-radius: 5px; padding: 10px 5px; font-size: 16px; } button{ float: right; margin-top: 20px; background-color: #1565c0; color: #fff; border: none; padding: 10px 30px; border-radius: 5px; font-size: 18px; } .result{ margin-top: 40px; display: none; } .result h3{ text-align: center; margin-bottom: 20px; font-size: 22px; } .wrapper{ display: flex; justify-content: space-around; } .wrapper span{ font-weight: 600; } .success{ color: #44b267; } .fail{ color: #e81c4e; }
After pasting the HTML and CSS code, Now have to create a third Javascript file with the named script.js. Paste the below code on it and save it. Again remember to give a .js extension to the javascript file.
//Random quotes api const quoteApiUrl = "https://api.quotable.io/random?minLength=80&maxLength=100"; const quoteSection = document.getElementById("quote"); const userInput = document.getElementById("quote-input"); let quote = ""; let time = 60; let timer = ""; let mistakes = 0; //Display random quotes const renderNewQuote = async () => { //Fetch content from quote api url const response = await fetch(quoteApiUrl); let data = await response.json(); quote = data.content; //Array of chars in quote let arr = quote.split("").map((value) => { return "<span class='quote-chars'>" + value + "</span>"; }); quoteSection.innerHTML += arr.join(""); }; //Logic to compare input words with quote userInput.addEventListener("input", () => { let quoteChars = document.querySelectorAll(".quote-chars"); quoteChars = Array.from(quoteChars); //Array of user input chars let userInputChars = userInput.value.split(""); //Loop through each char in quote quoteChars.forEach((char, index) => { //Check chars with quote chars if (char.innerText == userInputChars[index]) { char.classList.add("success"); } //If user hasn't entered anything or backspaced else if (userInputChars[index] == null) { if (char.classList.contains("success")) { char.classList.remove("success"); } else { char.classList.remove("fail"); } } //if user entered wrong char else { if (!char.classList.contains("fail")) { //increament and displaying mistakes mistakes++; char.classList.add("fail"); } document.getElementById("mistakes").innerText = mistakes; } //Return true if all chars are correct let check = quoteChars.every((element) => { return element.classList.contains("success"); }); //End test if all chars are correct if (check) { displayResult(); } }); }); //Update timer function updateTimer() { if (time == 0) { //End test if reaches 0 displayResult(); } else { document.getElementById("timer").innerText = --time + "s"; } } //Set timer const timeReduce = () => { time = 60; timer = setInterval(updateTimer, 1000); }; //End test const displayResult = () => { //Display result div document.querySelector(".result").style.display = "block"; clearInterval(timer); document.getElementById("stop-test").style.display = "none"; userInput.disabled = true; let timeTaken = 1; if (time != 0) { timeTaken = (60 - time) / 100; } document.getElementById("wpm").innerText = (userInput.value.length / 5 / timeTaken).toFixed(2) + "wpm"; document.getElementById("accuracy").innerText = Math.round(((userInput.value.length - mistakes) / userInput.value.length) * 100) + "%"; }; //Start test const startTest = () => { mistakes = 0; timer = ""; userInput.disabled = false; timeReduce(); document.getElementById("start-test").style.display = "none"; document.getElementById("stop-test").style.display = "block"; }; window.onload = () => { userInput.value = ""; document.getElementById("start-test").style.display = "block"; document.getElementById("stop-test").style.display = "none"; userInput.disabled = true; renderNewQuote(); }
That’s all after pasting the code your code will be successfully run. If you get any kind of error/problem in the code just comment or contact me on social media
Output Result
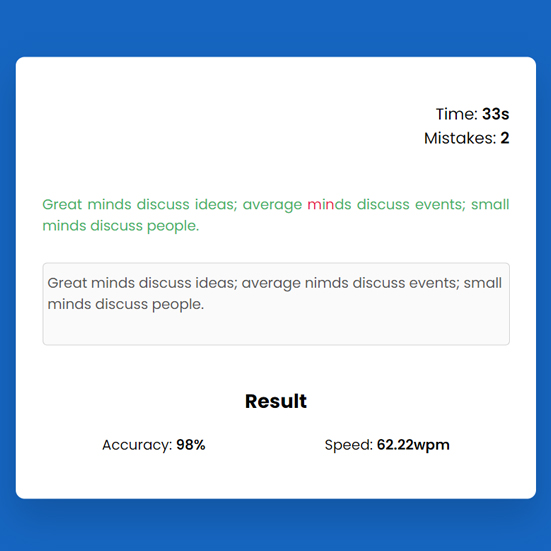
Written By | @narendra-chand |
Code By | Coding thai |
Code idea | @AsmrProg |